Suppose an array has 100 int elements. When you assign the first element of the array to v[100], the system creates a pointer to the first element of the array and sets v[0] to that value. Next, it assigns 100 to v[1], then 101 to v[2], and so on up to v[99]. The last element in the array is assigned 1010.
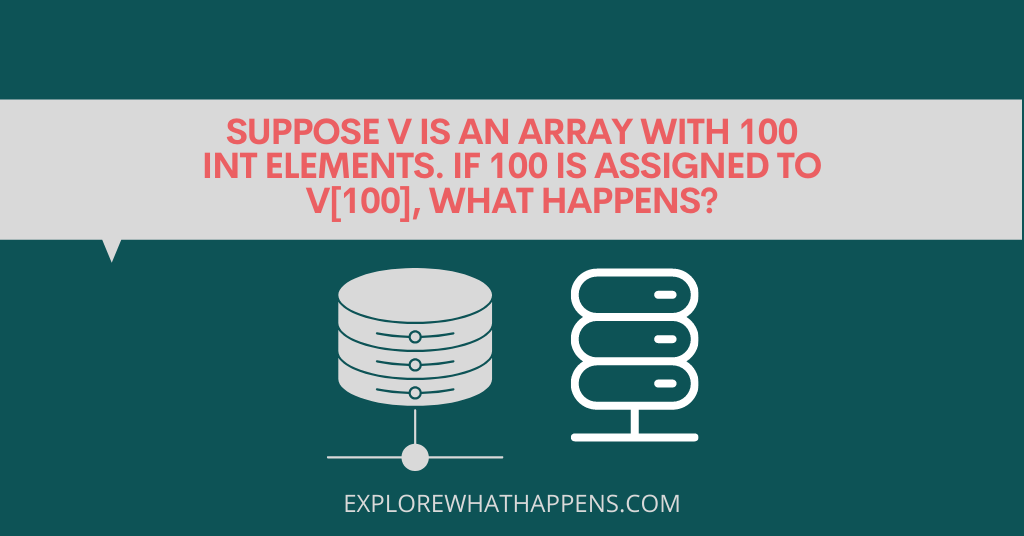
This would result in an out-of-bounds error, as v only has 100 elements.
If this is the first time that you have looked at arrays, then this will be a nice little project for you.
First of all, we will create a new array.
int v[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
As you can see there is an array of 100 int elements.
We will need to find the number of elements that are equal to 1.
We rely on the fact that arrays are sorted by default.
int index = 0;
foreach (int i) {
if (v[i] == 1)
index++;
}
How do we then find the number of elements that are equal to 1?
we assign an int index to v[], we loop through the array using a while loop.
we check every index to see if it is equal to 1.
If the index is equal to 1 then the index value is increased by 1.
Once the index is increased by 1, the current value of v[] is increased by 1.
Once the iteration is complete, the index value is checked again.
If the index is still equal to 1, then the loop continues.
If the index value is not equal to 1, then the loop is completed.
We now have the number of elements equal to 1.
It is possible to do this without using a loop.
int count = 0;
count = 1;
while (1) {
v[count] = 1;
count++;
}
This method will be faster but will be less accurate.